陣列的操作
some()、every()、find()、findIndex()、slice()、splice()
目標
- 由二組資料中,根據不同需求條件篩選出正確的資料
1 | - const people = [ |
實作要點
- 利用 some(),判斷 people 中是否有 19 歲以上的人。
- 利用 every(),判斷 people 中是否都 19 歲以上。
- 利用 find(),找到 comments 中 id 是 823423 的資料。
- 利用 findIndex(),找出 comments 中 id 是 823423 的索引值。
- 利用 splice()、slice 刪除 comments 中 id 是 823423 的資料。
實作
判斷 people 中是否有 19 歲以上的人
→ some()
1 | const ans0 = people.some(p => new Date().getUTCFullYear() - p.year >= 19); |

判斷 people 中是否都 19 歲以上
→ every()
1 | const ans1 = people.every(p => new Date().getUTCFullYear - p.year >= 19); |

找到 comments 中 id 是 823423 的資料
→ find()
1 | //find() 方法會回傳第一個滿足所提供之測試函式的元素值。否則回傳 undefined。 |

找出 comments 中 id 是 823423 的索引值
→ findIndex()
1 | const ans3 = comments.findIndex(comment => comment.id === 823423); |

刪除 comments 中 id 是 823423 的資料
→ slice()
- slice 為 淺拷貝(shallow copy),不影響原陣列資料(取得新的陣列資料)。
1 | const ans4 = comments.slice(0, ans3); //切ans3之前的 |

→ splice()
- splice 為 直接處理現有的陣列資料
1 | const ans8 = comments.splice(ans3, 1); //ans8就是刪掉的東西,沒留就不見了。 |
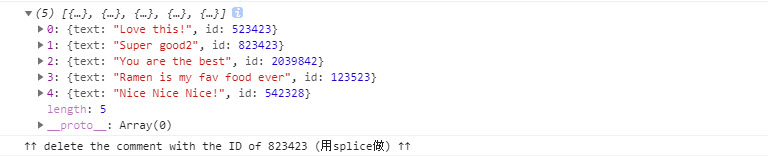
